Hello Swift - My first iOS app using SwiftUI
It took me some time. Fine, it took me a few years, but I finally got around to creating my first iOS app, using SwiftUI.
Created a simple single screen app that displays a random quote on the click of a button. A different quote each time you click that button. That’s it.
I’ve been reading James Clear’s fantastic book Atomic Habits last couple weeks, and loving it. I figured I would add some gems from the book, so each time you hit that button, you get a fresh dose of Atomic Habits.
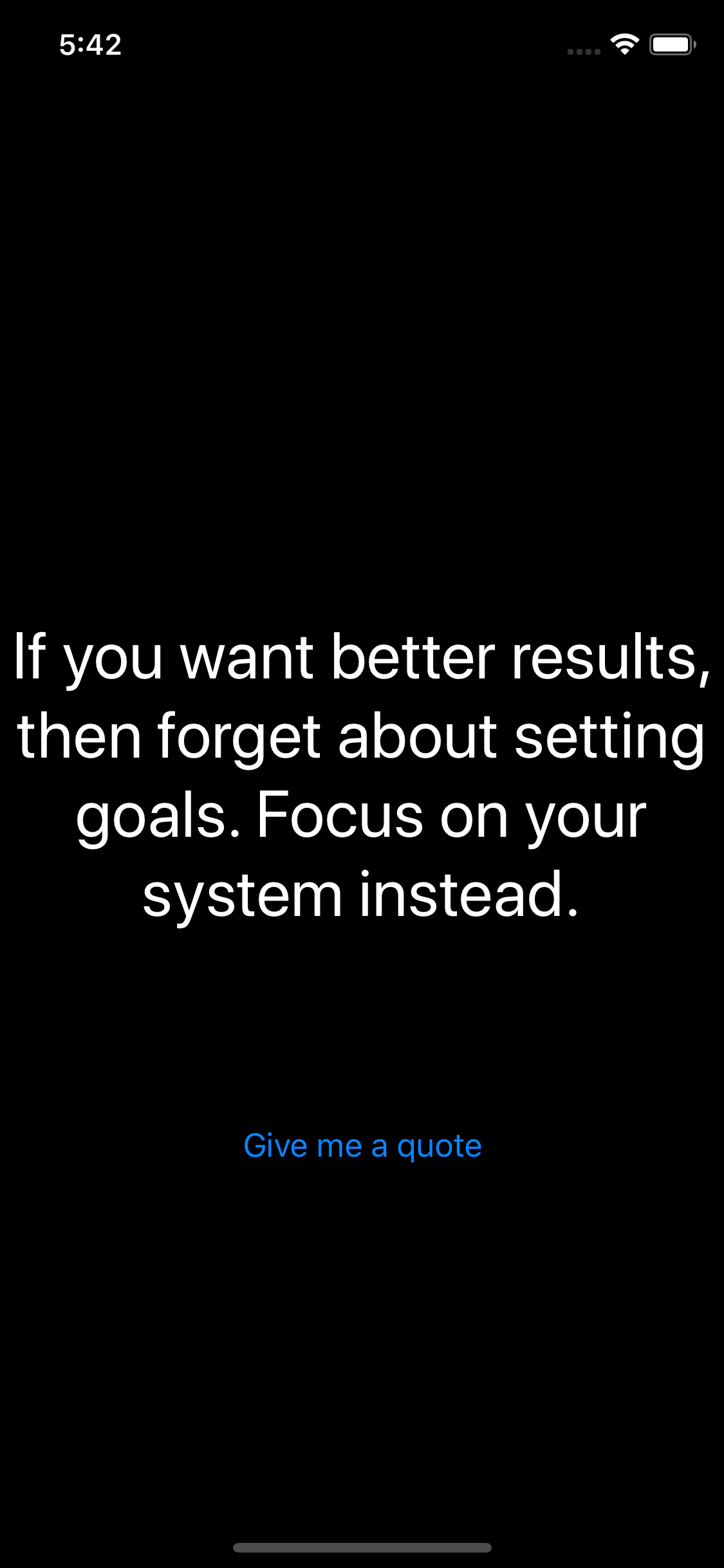
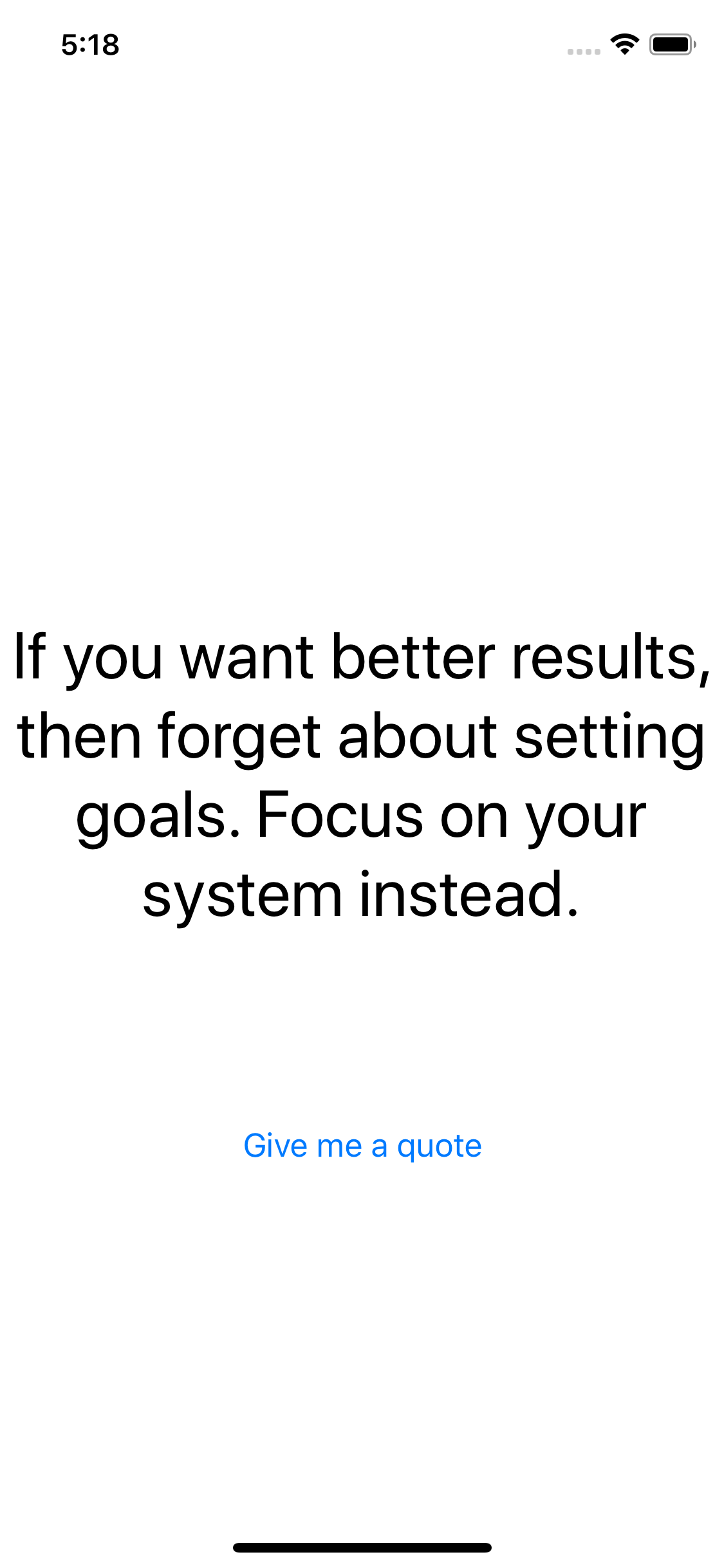
Steps
If you’ve never created an iOS app, I leave you with the basic steps, and the code that you can copy/paste. Thanks to SwiftUI, simply pasting this code will generate the view and the functionality for you.
- Open Xcode, and create a new project . File -> New -> Project
- Choose Single View App -> Next
- Give it a Name, for example - MyQuotesApp
- Leave the Language set to the default of Swift
- Leave the User Interface set to the default of SwiftUI
- Uncheck the boxes for Core Data, Unit Tests, and UI Tests
- Select the file ContentView.swift in the project navigator on the left.
- Paste the code below inside this file.
cmd
+s
to save the filecmd
+r
to run the code- That should open the simulator and display the app
Source Code
// Inside ContentView.swift
import SwiftUI
struct ContentView: View {
//define the variable quoteText as @State, so we can track any changes to it, and update our display accordingly
@State var quoteText = "Quote goes here"
var body: some View {
VStack {
Text(quoteText) //Text object to hold the quote
.font(.largeTitle)
.multilineTextAlignment(.center)
.padding(.vertical, 100.0)
Button(action: {
//generate random number
let index = Int.random(in: 0..<quotes.count)
//pull a quote from the array using a random index number
let quote = "\(quotes[index])"
//update the state for the varaible quoteText
self.quoteText = quote
print(quote) //prints to the console
}) {
Text("Give me a quote")
}
}
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
let quotes = [
"You do not rise to the level of your goals. You fall to the level of your systems.",
"You should be far more concerned with your current trajectory than with your current results.",
"Goals are good for setting a direction, but systems are best for making progress.",
"Problem #1: Winners and losers have the same goals.",
"Habits are the compound interest of self-improvement",
"If you want better results, then forget about setting goals. Focus on your system instead.",
"Professionals stick to the schedule; amateurs let life get in the way.",
"When you can’t win by being better, you can win by being different.",
"Every action you take is a vote for the type of person you wish to become.",
"Success is the product of daily habits—not once-in-a-lifetime transformations."
]
Next, I want to get that data dynamically by calling an API. That's for another post.